python class decorator with arguments
Return a b add 10 20 Code. Functions can be returned from a function.
Understanding Args And Kwargs Arguments In Python
The first argument is the instance so it reads the attribute off of that.
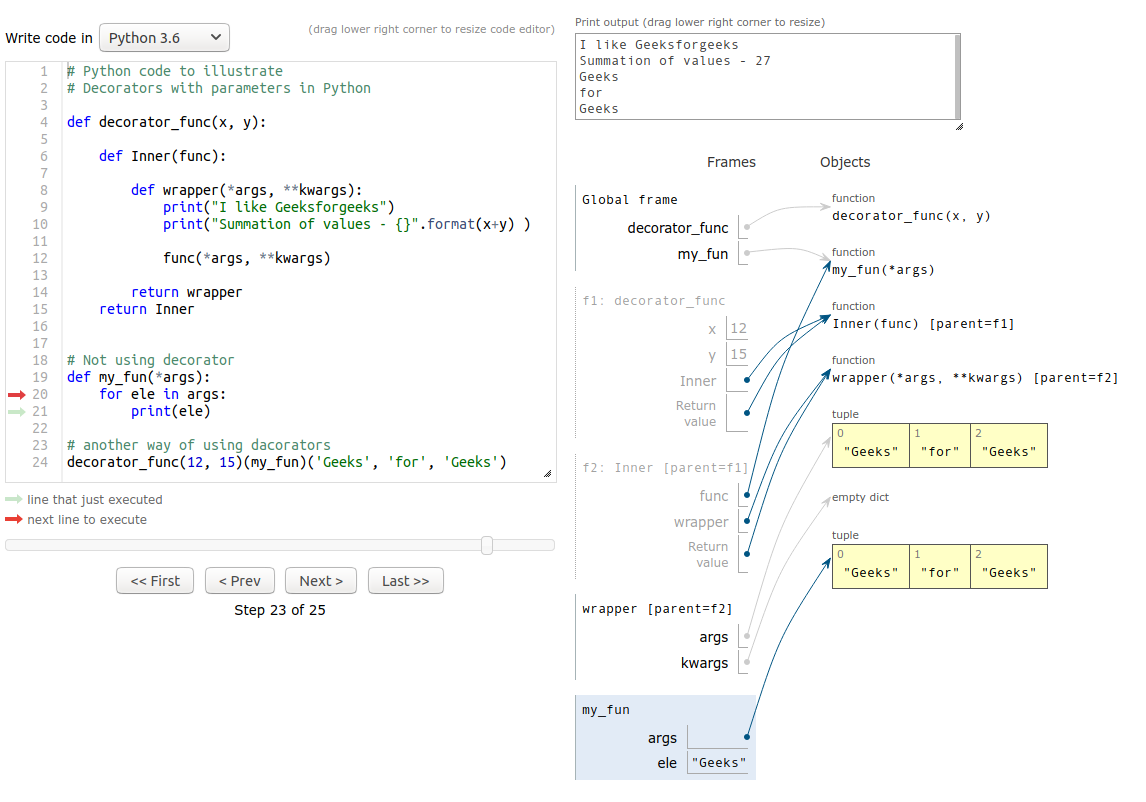
. Selffunction args kwargs MyDecorator. Functions can be referenced to a variable. The logic in the wrapper checks if the name argument for the decorator is simon and if it is it runs the decorated function.
Decorators can have arguments passed to them. Print call my_function args kwargs return 3. Print __init__a kw def __call__ self a kw.
Wrapsfunc def wrapperargs kwargs. It is one of the most powerful features of Python. Args will take an unlimited number of arguments of any type such as 10 True or Brandon.
From functools import wraps import time def sleepsecondsNone. Def __init__self function max_hits10 timeout5. Inside of that is the wraps decorator on the wrapper function like I showed you in the previous lesson.
Decorators with parameters is similar to normal decorators. For _ in range times. Result fn args kwargs return result return wrapper return decorate.
Arguments With Class Decorators in Python. Self args 0. PrintInside wrapper function argement passed args 0url return f args return wrapper.
Def __call__ self args kwargs. Function implementation The above code is equivalent to def func_name. You can pass in the attribute name as a string to the decorator and use getattr if you dont want to hardcode the attribute name.
Python functions can be treated as supreme citizens as objects. Selffunction function selfmax_hits max_hits selftimeout timeout selfcache def __call__self args. 0049 As a decorator is a closure generator that takes a data function the first inner function is exactly that.
Selfparam_foo param_foo selfparam_bar param_bar def __call__self func. In this code the decorate function is a decorator. The syntax for decorators with parameters.
A decorator in Python is a function that takes another function as an argument and extends its behavior without explicitly modifying it. This can be applied to a regular decorator in order to add parameters. Def __init__self param_fooa param_barb.
Def wrapper self args. Python decorator are the function that receive a function as an argument and return another function as return value. Both types work fine but the class decorator that can take an argument is more flexible and efficient.
Follow this answer to receive notifications. To add an argument to a decorator I ended up having three levels of functions see here and here. Let us see both cases one by one.
Def layer args kwargs. Return dec f args kwargs return repl return layer. Def __init__ self function.
The decorator intercepts the method arguments. Wraps fn def wrapperargs kwargs. Decorators are a very powerful and useful tool in Python because it allows users to modify the behaviour of function or class.
You could instead use a decorator factory that can take optional max_hits and timeout arguments and returns a decorator. Star 5 def adda b. The following illustrates how to use the star decorator factory.
Print __call__a kw MyClassDecorator 123decorator configuration def my_function args kwargs. How to Add Arguments to Decorators in Python. Function implementation func_name decoratorparamsfunc_name.
A class decorator has two types. Call a function a number of times def decoratefn. Print getattr self attribute.
The star is a decorator factory that returns a decorator. Functions can be passed as an argument to other functions. It accepts an argument that specifies the number of characters to display.
As a decorator is a function it actually works as a regular decorator with arguments. If it isnt it raises an error. PrintSleeping for secondsformatseconds timesleepseconds if seconds else 1 return.
One accepts arguments and the other does not. Here the code returning the correct thing. Def __init__ self a kw.
The assumption for a decorator is that we will pass a function as argument and the signature of the inner function in the decorator must match the function to decorate. Whatever logic your decorator is supposed to implement goes in here printpre action baz printselfparam_bar including the call to the decorated function if you want to do that. To solve this you need to create decorator outside the class that gets only function and that functions first parameter will be self.
In order to use class decorator with argument args and kwargs we used a __call__ function and passed both the argument in a given function. It has several usages in the real world like logging debugging authentication measuring execution time and many more. This time we will look at a scenario where we define a function add_num that adds two numbers.
To add arguments to decorators I add args and kwargs to the inner functions. Def __init__ self url.
What Is Dict And List Comprehensions In Python And How To Use Them Python Tutorials Point Comprehension Being Used Python
Decorators With Parameters In Python Geeksforgeeks
Decorators With Parameters In Python Geeksforgeeks
Class Based Decorators Make Your Python Code Classy Idego Group
Python Property Decorator Python Property Journaldev
How To Use Python Property Decorator Askpython
Python Decorator With Arguments Python Decorator Tutorial With Example Dev Community
Python Decorators In 15 Minutes Youtube
Python Decorators Explained If You Ve Spent Even A Little Bit Of By Matt Harzewski The Startup Medium